Ideas For Docker up.ps1 Scripts
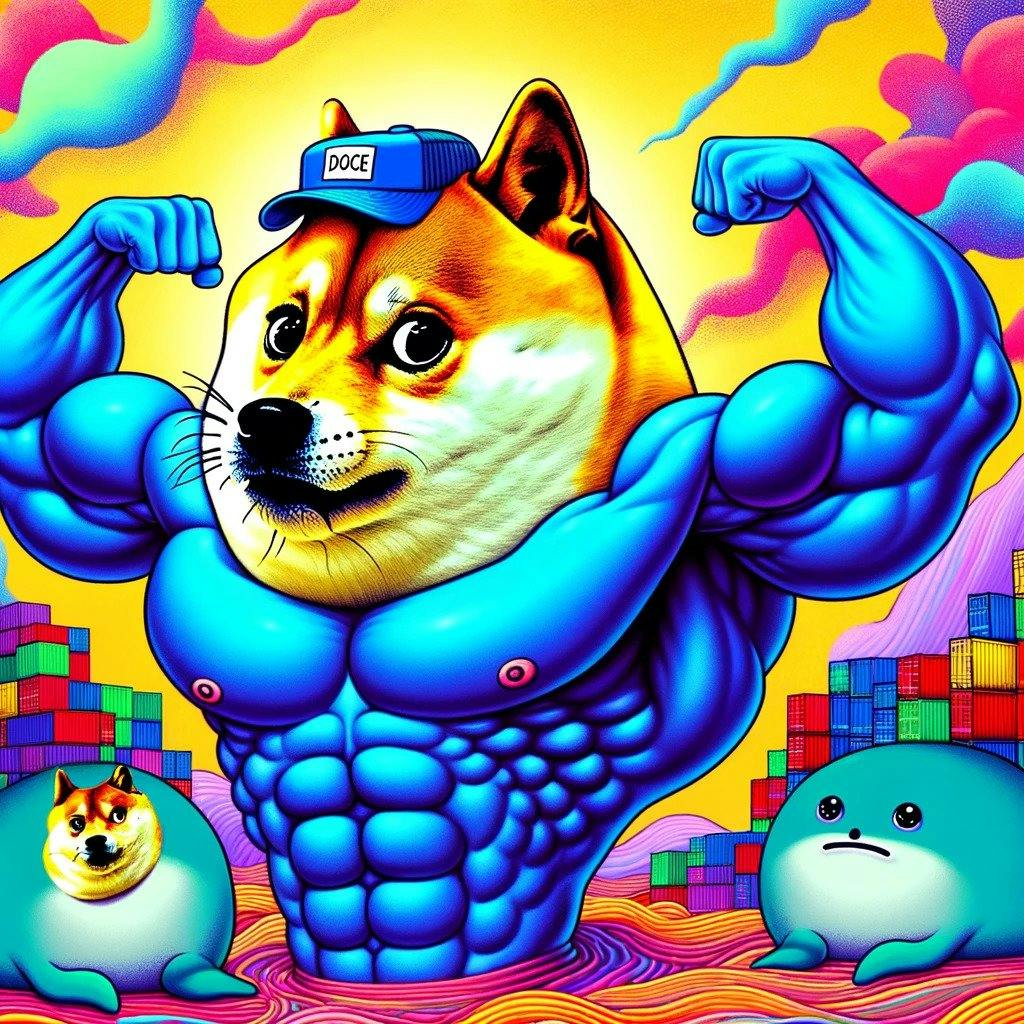
Introduction
When working with Docker, it's easy to get lost in the sauce. There are a lot of moving parts (despite what the marketing says), and it's easy to forget what you need to do to get things up and running. This is especially true if you're switching between projects, some of which use Docker and others that don't.
A Perfect World
Imagine a perfect world where it takes only one command to have a fully functional Sitecore instance running in Docker:
git clone https://github.com/user/sample-project.git && cd sample-project && powershell -File up.ps1
Sound too good to be true? Of course it is!
However, we can get close to this with a solid up.ps1
script. Is it a good idea? Maybe not. But it's fun to think about.
Current Example
Let's see what the latest and greatest example is from Sitecore. This repo is the current example project for XM Cloud and is used by the Sitecore MVP site: https://github.com/Sitecore/XM-Cloud-Introduction/blob/main/up.ps1
The key features and functions are:
- Option to run against edge, vs local, thus affecting the run of Traefik and host containers.
- Build images. Update the XM Cloud base image and build all Sitecore Docker containers.
- Index and push items.
- Open the site and CM in a browser.
- Environment variable loading and validation.
Overall, it's a relatively simple script.
Maximalist Up Script
Some have argued that up.ps1
should do as little as possible. There is something to be said about keeping things simple and letting things fail. YAGNI, the best code is no code, let it crash, etc. However, I would argue that the up.ps1
scripts I have seen don't do enough (or don't exist at all). In order to explore this concept, I will take the idea of writing a maximalist up script to the extreme, listing a series of ideas for what the script could assist with.
Qualities of a maximalist up script:
- Automatically do most things that you would otherwise need to do manually.
- Detect specific errors which are commonly encountered and overcome them in real time or provide helpful error messages.
- Should be able to be run multiple times without issue.
- Functionality is configurable via environment variables and/or script flags.
Here's the list of ideas:
- Check that the .env file exists and is populated and run
init.ps1
if needed. - Check if a new version of Docker is available.
- Check that the necessary ports on the host machine aren't already in use.
- Check that IIS is shut off.
- Check that the Docker service / Docker Desktop are installed / running. If not, start them.
- Check that the Dependencies are OK.
- Check that the database server is reachable (if running DBs on host machine).
- Check that the script has been run with the necessary permissions.
- Check validity of custom Sitecore configs to ensure that they are well-formed and don't contain any obvious errors. If bad configs make their way into your containers, it can take a while to track down the issue.
- Check key environment variables.
- Show current version of node, npm, dotnet, PowerShell, Docker, docker compose, etc.
- Show CPU, memory, disk space, etc. to identify potential runtime errors or performance bottlenecks.
- Show the host name of the machine running Docker Desktop.
- Restart Docker Desktop as doing so can fix all kinds of issues.
- Run git commands (pull, etc.).
- Build containers.
- Start the containers.
- Open Sitecore in a browser.
- Open the rendering host in a browser.
- Log in to Sitecore.
- Sync serialized items to Sitecore.
- Populate Solr schema.
- Rebuild indexes.
- Run automated smoke tests to ensure that key functionalities are working as expected.
- Warm up the site by making requests to key pages.
- Call ChatGPT to do... ???
- Print a funny message similar to Sitecore PowerShell Extensions' script execution messages.
- ASCII art.
- Choose your own adventure game.
- Call your parents.
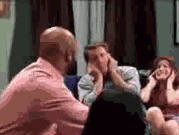
Example Script
Below is an example up.ps1
script that does some of the above. I don't take credit for much of it; it's mostly a soup of other code and ideas I have found online.
Conclusion
Personally, I have saved much time and frustration by writing a solid up.ps1
script, so I approve of this post. 😉
If you have any interesting functionality in your up.ps1
scripts, please get in touch!
Keep up.ps1ing your game,
-MG