Tips for Forms Implementations
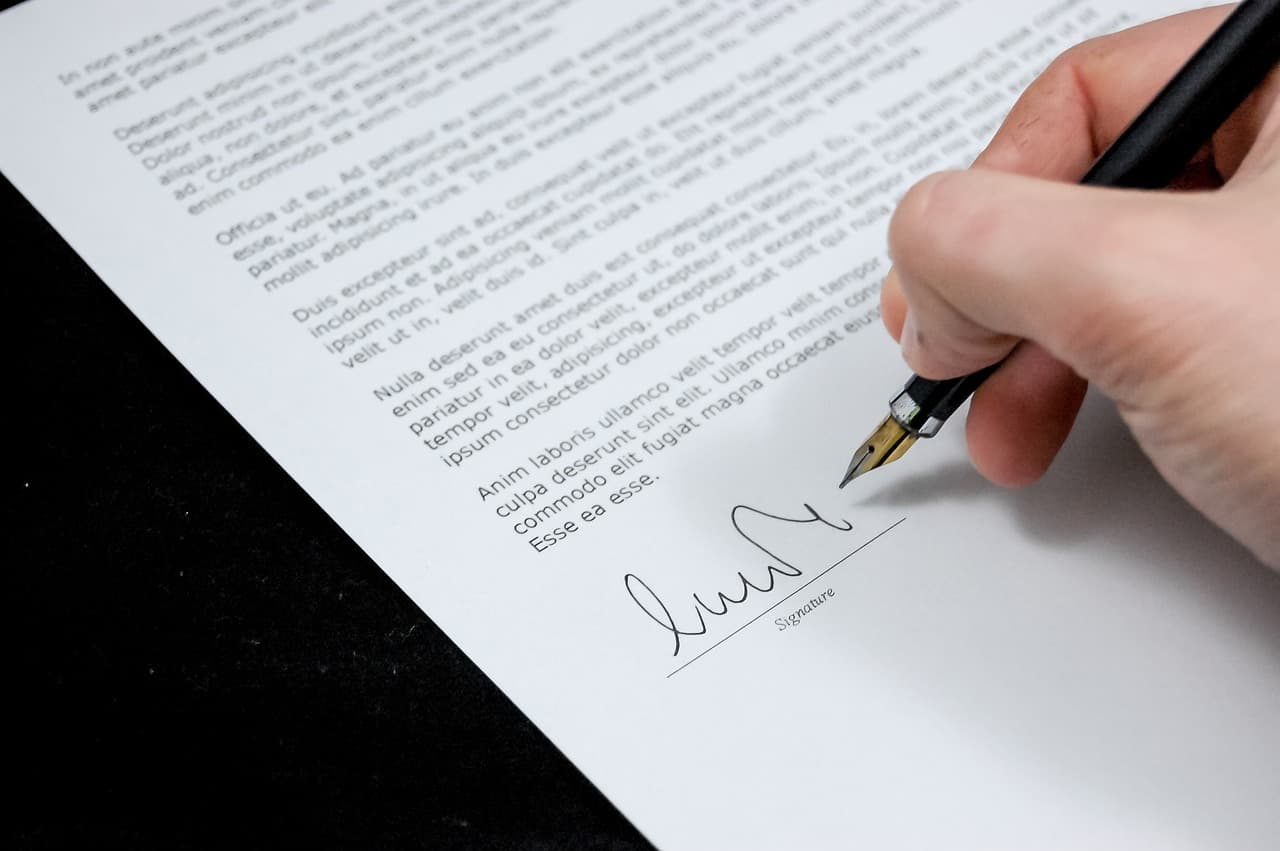
Index
- Sitecore Forms History
- Take Forms Implementations Seriously
- XConnect + Forms Integrations and Submit Actions
- Testing Forms on CM
- Exception Alerting
- File Attachments
- Form Validation
- Multi Page Forms
- Implement Captchas From Day One
- Honey Pots
- General Error Handling
- Submission Confirmation
- Logging & Writing to DB
- Long Forms and User Sessions
- Email Addresses
- Other Considerations
- Conclusion
Sitecore Forms History
Forms have always been a hot topic, both on the web in general, and in Sitecore. As of Sitecore version 9, Sitecore natively shipped with new Forms functionality as a successor to Web Forms for Marketers. Everyone was eager to use the new functionality, but quickly ran into limitations. For a while, Sitecore Forms Extensions, a custom module, did a great job of filling in many of the missing pieces. Over the years, the native Sitecore Forms has improved, and is now a solid OOB option.
However, with the advent of XM Cloud, we're at the new frontier of forms again, because Sitecore Forms aren't supported in XMC. I believe we are going to see all kinds of community driven solutions pop up, as well as the adoption of dedicated third party solutions.
Over the years I have noticed quality issues with regards to both custom and OOB Sitecore Forms implementations. This post will help you provide more realistic estimations and additional client satisfaction regarding forms projects.
Note: this post is intentionally extreme and is quite paranoid and thorough in order to help you consider all possible issues. You can (and probably will) take a more relaxed approach, but I recommend that you at least consider everything mentioned here.
Take Forms Implementations Seriously
Ponder this:
One of the worst things that can happen on a website is when form submissions fail (especially when they fail silently). It only takes one critical failed form submission to erupt in chaos. Form issues are one of the easiest ways to lose your client's trust, so don't screw them up.
Forms implementations are often under-budgeted and complexity is often underestimated. Further, clients often underestimate the importance of forms, and the negative impact that a poor form implementation form can have on user experience, and, more importantly, on their brand reputation.
As an implementation partner, it is your responsibility to understand the intricacies of forms and to educate the clients on why they should allocate sufficient budget to ensure their forms are bulletproof.
Make sure your client understands the importance and complexity of forms. Think through all the scenarios and edge cases carefully.
Any time that you have custom code as part of a form submission, it is important to consider all scenarios and edge cases. The goal is to prevent any of the following:
- Silent form submission failures (user is not made aware of the failure)
- Non-silent form submission failures that result in a poor user experience on the front end
- Fatal infrastructure errors that go unnoticed (which is common when your forms integrate "external" systems such as SMTP and XConnect)
- Inability for content authors to preview and test forms
XConnect + Forms Integrations and Submit Actions
One example of when forms implementations can go wrong is when you are integrating with XConnect. Consider this simplified case in which you have a custom submit action that identifies users based on their form data:
The call to this can throw exceptions:
This can happen when the xconnect instance is unreachable, or when there are SSL certificate issues, which happens more commonly than you might think.
Example errors:
Not wrapping this code block in a try-catch (or not handling the error elsewhere) can cause form submissions to fail entirely, resulting in unexpected states on the front end. When these errors occur, handle them and consider setting up real time alerts, as this is a critical issue.
Built-in submit actions in Sitecore Forms (that I have seen) all perform a check to see if there was an error with the submission, or an error in the previous submit action. If there is an error, they will not execute. This is a good practice to follow, and you should consider doing the same in your custom submit actions, while keeping implications in mind. Also, decide which custom submit actions are critical or not.
Testing Forms on CM
Did you know that XConnect is effectively disabled when you are logged into Sitecore? This is done to prevent testing data from polluting the real analytics data. Are you accounting for this scenario if a content author wants to test a form in preview mode?
You can also test on CM with Explore mode in Experience Editor.
Exception Alerting
Make use of tools such as Sentry and Azure Alerts to be notified when exceptions occur. This is a critical part of any production application, and should be a top priority.
File Attachments
File attachments are a common feature in forms. However, they are often not implemented correctly. What happens when a user goes to upload a file and then hits cancel? What happens if the user wants to delete a file? What happens if the user uploads a file that is too large, or uploads an invalid file extension?
- Number of files
- File saving location (public? private?)
- File size limits
- File type limits
- File name limits
- File name sanitization / OS friendliness / path length limits
- File name uniqueness
- Max POST size
- Cleanup process
- Disk space monitoring
- Packet loss (the larger the size, the higher the chance). The only way to address this is to have a progressive upload before the form submission (think Gmail file attachments). This will require a good amount of code and can only be done if the page is only accessible by identified users.
- Single POST vs multi POST
Form Validation
This goes without saying: implement both client and server side validation and validate the hell out of your forms.
Pro tip: using Sitecore Forms, understand that it is quite easy for content authors to forget to set max length validation on text fields.
Multi Page Forms
Consider splitting large forms up into multiple pages for a better user experience. In Sitecore Forms, you can specify an additional form page to be used as a success page (via form submit action).
Implement Captchas From Day One
Clients often don't realize how crucial these are until they start getting bombarded with SPAM. Honeypots aren't good enough (though they are recommended). Implement the Captcha.
Honey Pots
Honey pot implementations need to be implemented correctly:
- Need to have accessibility metadata to prevent real users from filling out the field
- Check on value should be a check on key
- Failure should not be reported back to the end user
General Error Handling
What happens when your form fails to send an email? What happens when your form fails to save to the database? What happens when your form fails to save to xConnect? What happens when your form fails to save the data?
Are you being alerted? Is the end user made aware of an issue having occurred? Is it clear to them that they should try again? Is it clear to them that they should contact you another way? Is there a way for them to save their form progress?
Submission Confirmation
When a user submits a form, it should be very clear that it was received. Often times, success messages appear as single lines of grey colored text below the submit button. Bad UX.
Consider emailing the submitter their own submission data. This is a great way to ensure that they have a copy of their submission, and that they can easily refer back to it in the future. It also lets them know that their submission was successful. However, this does add more complexity.
Logging & Writing to DB
When logging form submissions, be careful not to log any sensitive data. Printing user info in the logs can be a privacy risk, depending on your situation. Also, logging is not a silver bullet. Logging can fail.
Writing to databases is also not impervious to issues. Consider worst case scenarios or edge cases such as unexpected increases in latency.
Long Forms and User Sessions
Here's a ghastly scenario: imagine that users must log into your site. On one of the pages, there is an important and long/comprehensive form. This form communicates information that is highly important.
Perhaps it's a form where you want to report professional misconduct, where you must provide lengthy explanations and extensive supporting documentation. The form takes some time to fill out. Long enough, even, that users may pause partway through and come back to finish it later.
Ask yourself: what happens when their session expires while they are away?
Email Addresses
If you are sending any kind of email as a submit action, or even collecting emails purely for informational purposes, consider this:
- Strict email validation. Validating emails via code (regular expressions) is impossible as it requires multiple layers of checks and constant adjustments as new specifications are introduced. This is why most things that rely on email require you to validate your email via an actual email send step. The only way to prevent user errors with certainty is to validate their email before the user submits a form. This will require one of the following:
- User registration and sending verification emails
- Use social login to verify email
- Bad email addresses (resulting in SMTP bounce)
- Blacklists
- SPAM filters
Other Considerations
- Network availability / speed / packet loss
- Browser compatibility
- Firewall rules. You may want increased security posture on pages containing forms (remember to add exceptions for well known crawlers as as
googlebot
) and certainly the POST routes themselves. - XSS (can be triggered by what users enter, even if they are not malicious)
- DDOS protection
- Disaster recovery -- what happens if your server goes down? What happens if your database goes down? What happens if your email server goes down? What happens if your XConnect server goes down?
Conclusion
This is all about ensuring you and your client understand the importance and complexity of forms, and to adjust budgets accordingly.
Now, go forth and build forms that are slightly less bad than the other ones out there!
-MG